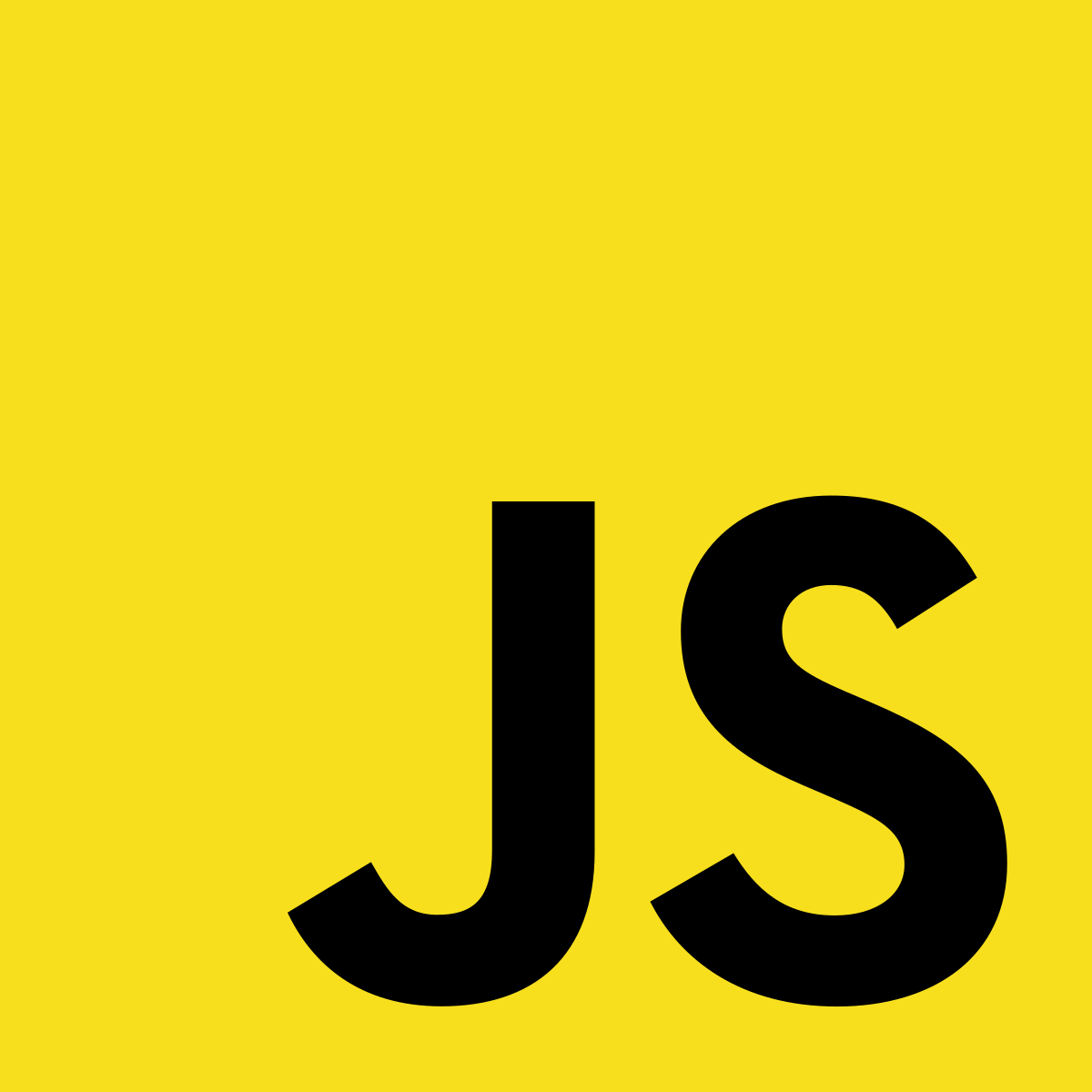
JavaScript Language Essentials for Coders
Are you a coder who wants to boost your web development skills? JavaScript is your answer. It’s key for creating interactive websites and working on the front end. But what’s so great about JavaScript for us coders? How does it make better code and fit well with different browsers? We’re going to explore JavaScript’s key points to show its true power.
Table of contents
Key Takeaways:
- JavaScript is a must-know language for web development and front-end coding.
- It enables the creation of interactive websites and dynamic content.
- JavaScript optimizes code and ensures browser compatibility.
- Understanding variables, operators, conditionals, and functions is essential for harnessing the full potential of JavaScript.
- Mastering JavaScript elevates your web development skills and empowers you to build engaging websites.
Introduction to JavaScript Language
JavaScript is a versatile programming language used to make websites interactive and dynamic. It lets developers create engaging user experiences. They can do this with dynamic styling, animations, and interactive elements like buttons and forms.
One strength of JavaScript is its compatibility with browser APIs. These APIs help in numerous ways, from changing HTML elements to adding video streaming and 3D graphics.
JavaScript allows developers to use third-party APIs for more website features. For instance, they can add comments or social media sharing using APIs from sites like Disqus or Facebook.
JavaScript makes development easier through many frameworks and libraries. These tools offer pre-built components that fast-track website and application creation.
Overall, JavaScript gives developers the power to create lively web experiences. It combines programming abilities, browser APIs, third-party APIs, and development tools.
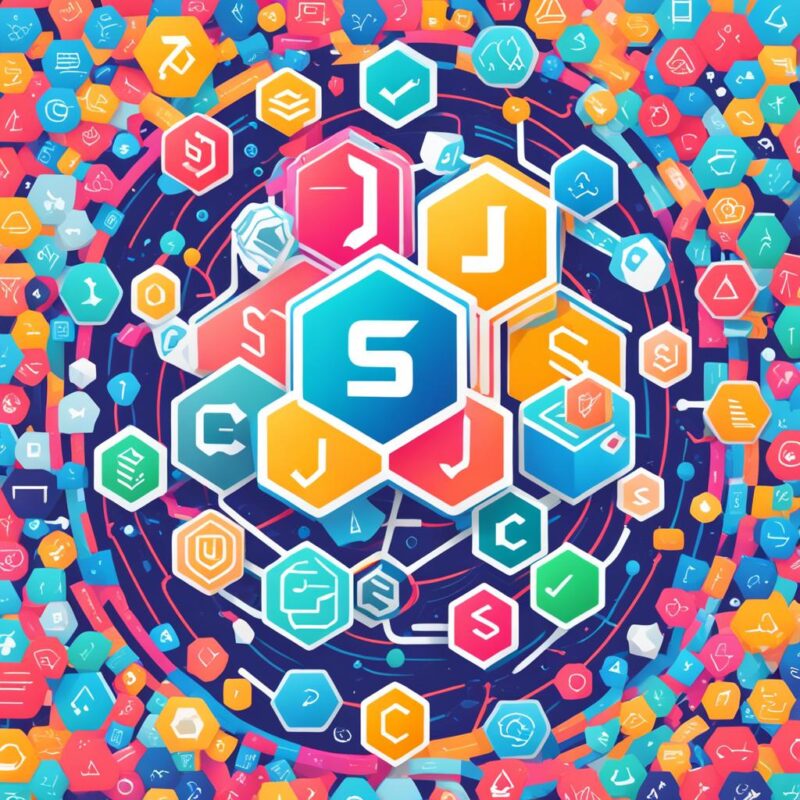
JavaScript Language Features
Here are some key features of JavaScript:
- It makes web pages interactive, responding to user actions.
- Developers can change HTML element styles dynamically.
- It supports creating animations for a better user experience.
- JavaScript uses browser APIs to interact with devices.
- It lets developers add third-party APIs for more features.
- There’s a wide variety of frameworks and libraries available.
Javascript Language Benefits
Using JavaScript comes with several advantages for web developers:
- It enables the creation of more engaging web interfaces.
- Developers can enhance websites with browser APIs and APIs from content providers.
- Time is saved using frameworks and libraries, reducing development effort.
- JavaScript works across all major browsers, ensuring applications are compatible.
- Its versatility allows for building scalable applications that can grow with time.
Getting Started with JavaScript Language
Let’s start by making a simple Hello world example in JavaScript. It’s an easy task that helps you learn how JavaScript code looks and works. First, include a <script>
tag in your HTML page to add the JavaScript code.
<script>
document.getElementById("myHeading").innerHTML = "Hello, world!";
</script>
In the example, we’re using JavaScript to change a webpage. The code uses the DOM API. It finds the heading with the ID “myHeading” and changes its text to “Hello, world!”.
It’s best to put your JavaScript code near the end of your HTML file, right before the closing </body>
tag. This order helps ensure your code runs properly after the page loads.
Benefits of DOM Manipulation
Working with the DOM in JavaScript has several advantages for developers. Here’s why it’s great:
- Dynamic updates let you change your website’s look instantly.
- User interaction means your page can respond when visitors do things like clicking or typing.
- Visual effects are easy, thanks to JavaScript, making your site more appealing.
- You can manage data, like changing or removing text on your pages.
So, with basic JavaScript and DOM principles in hand, you’re set to explore more of this exciting language.
Term | Description |
---|---|
Hello world example | A simple program that displays the phrase “Hello, world!” and is often used as a starting point for learning a new language. |
Script inclusion | The process of adding external JavaScript files into an HTML page via the <script> tag. |
DOM manipulation | The way JavaScript interacts with web pages, lettings you change and adjust what users see and do. |
Understanding Variables in JavaScript Language
In JavaScript, variables are like boxes. They store different kinds of information like strings, numbers, Boolean values, arrays, and objects. This makes it easy for developers to handle info in their code.
Developers use different keywords to create variables, such as let, const, and var. Each keyword works a bit differently, affecting how the variable can be used.
The let keyword is great for temporary storage. Variables with let can only be used in the part of the code where you put them. This keeps things clear and avoids confusion.
The const keyword is perfect for things that never change. Once you use const to set a value, that value stays the same. It’s a helpful tool for keeping your code stable.
The var keyword is the original way to make variables in JavaScript. Unlike let and const, you can use a var variable anywhere in your code. This can be both good and bad, depending on what you’re working on.
Data Types in JavaScript:
JavaScript has different data types for different jobs. Here is what it can work with:
- Strings: These hold text and are shown in either single or double quotes.
- Numbers: For anything that needs to count or measure, like ages or prices.
- Boolean values: They show if something is true or false, that’s it.
- Arrays: Let you group lots of stuff together in one place, easy to get to each item.
- Objects: More complex data, organized by pairs of keys and values.
Grasping these concepts helps developers create amazing websites. With the right use of variables and data types, programs become flexible and engaging.
Table: Variables in JavaScript
| Variable Keyword | Description |
|——————|——————————————————————————–|
| let | Declares block-level variables accessible within the scope they are defined. |
| const | Declares variables with values that cannot be changed once assigned. |
| var | Older way of declaring variables; not recommended due to unexpected behaviors. |
Working with Operators in JavaScript Language
JavaScript is a strong language that comes with many operators. These operators are key for doing math, making decisions, and guiding how a program runs.
Arithmetic Operators
In JavaScript, we have operators for math like addition (+), subtraction (-), multiplication (*), division (/), and modulus (%). We use these when dealing with numbers.
Assignment Operators
There are assignment operators too, such as += and -=. They help us change a variable’s value by adding or subtracting. It’s great for updating values easily.
Comparison Operators
For checking values, JavaScript gives us comparison operators. They include ==, ===, , =, and !=. We use these in if statements and loops to see if conditions are met.
Logical Operators
To mix and negate conditions, use logical operators. These are && for AND, || for OR, and ! for NOT. They’re vital for complex checks.
“JavaScript operators are vital for dynamic apps. They allow for calculations, data comparison, and flow control. Mastering these is key to JavaScript success.”
JavaScript’s operators are crucial for creating powerful code. They let us work with numbers, change variables, compare values, and check conditions. They’re essential for the web development world.
Operator | Description | Example |
---|---|---|
+ | Addition | let result = 2 + 3; |
– | Subtraction | let result = 5 – 2; |
* | Multiplication | let result = 3 * 4; |
/ | Division | let result = 10 / 2; |
% | Modulus (Remainder) | let result = 7 % 2; |
+= | Addition Assignment | let x = 5; |
-= | Subtraction Assignment | let x = 10; |
== | Equal to | if (x == 5) { … } |
=== | Strictly Equal to | if (x === 5) { … } |
&& | Logical AND | if (x > 0 && x |
|| | Logical OR | if (x === 0 || x === 10) { … } |
! | Logical NOT | if (!condition) { … } |
Conditional Statements in JavaScript Language
JavaScript has powerful tools for controlling a program’s flow. This is based on certain criteria. These tools include if, else, and switch statements. Let’s dive into what each does:
If Statement
The if statement runs a code block only if the condition is true. It starts with a set condition in brackets. Then, what to do if it’s true is put in braces. Here’s how it looks:
if (condition) { // code to be executed }
Else Statement
The else statement comes after an if statement. It lets developers say what happens if the condition isn’t met. Here’s an example:
if (condition) { // code to be executed if condition is true } else { // code to be executed if condition is false }
Switch Statement
The switch statement handles many conditions more simply. It checks one variable’s value against several cases. Then, it runs the block of code for the correct case. Here’s how it works:
switch (variable) { case value1: // code to be executed if variable equals value1 break; case value2: // code to be executed if variable equals value2 break; default: // code to be executed if variable doesn't match any case }
These are key for making decisions in JavaScript. They let programmers guide their programs based on different situations. This makes your code adaptable and quick to react.
Functions in JavaScript Language
Functions are key in JavaScript, grouping code we can run whenever we want. There are several types, like function declarations, function expressions, and arrow functions. Now, we’ll look at each one closely.
Function Declarations
Function declarations are a basic way to make functions in JavaScript. When you use the function
keyword, a function’s name, and parentheses, you’ve defined one. With this, you can call the function anywhere in your code.
<pre>
<script>
function greet() {
console.log("Hello, world!");
}
greet(); // Output: "Hello, world!"
</script>
</pre>
The greet()
function we see above uses the function
keyword. Calling this function writes “Hello, world!” on the screen.
Function Expressions
Function expressions are functions you assign to a variable. This concept lets you treat them as values. They can be either named or nameless.
<pre>
<script>
const sayHello = function() {
console.log("Hello!");
}
sayHello(); // Output: "Hello!"
</script>
</pre>
The example above shows a function expression in action. We use the sayHello
variable to call it. This prints “Hello!” to the console.
Arrow Functions
Arrow functions provide a short method to make functions and are popular in today’s JavaScript. They simplify syntax and automatically manage the function’s scope.
<pre>
<script>
const multiply = (a, b) => {
return a * b;
}
console.log(multiply(2, 3)); // Output: 6
</script>
</pre>
The code above defines an arrow function named multiply
. It accepts two numbers, multiplies them, and shows the result. When we call it with 2 and 3, it prints 6.
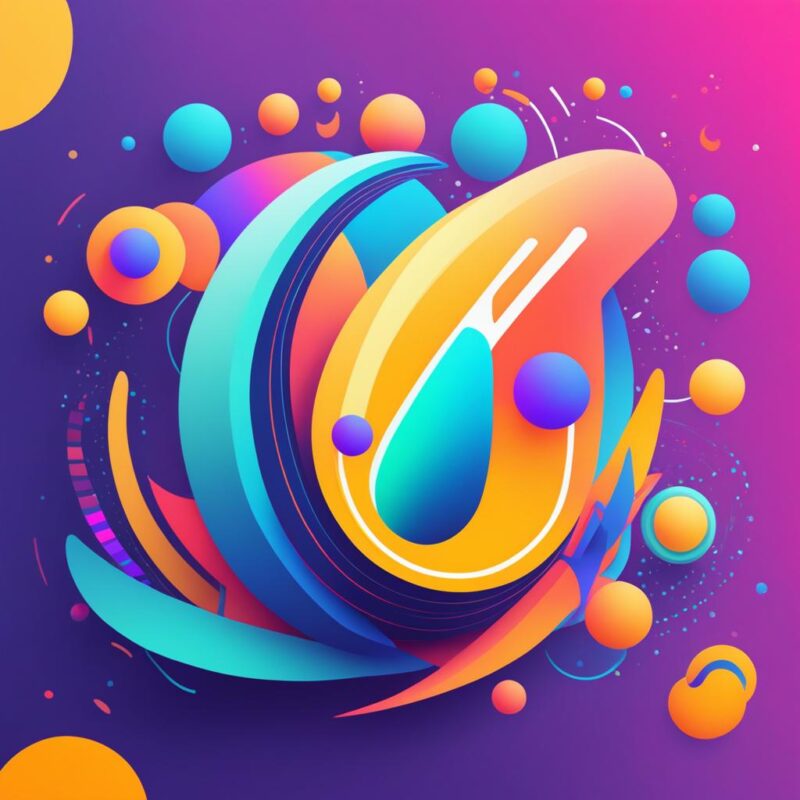
Function Type | Description |
---|---|
Function Declaration | Defines a named function that can be called by its name. |
Function Expression | Creates a function as a value assigned to a variable, which can be invoked using the variable name. |
Arrow Function | Provides a concise syntax for creating functions and is often used in modern JavaScript development. |
Conclusion
JavaScript is key for web development. It’s flexible and powerful, helping to build dynamic websites. It uses things like variables and functions to create cool web apps.
With JavaScript, developers add life to their projects. They can make websites that users love to interact with. This makes web pages work better for everyone.
Learning JavaScript is smart for anyone in web development. It’s very important because it’s everywhere online. With JavaScript, you can make amazing web projects for people to enjoy.
FAQ
JavaScript is an important language for web and front-end development. It makes websites dynamic and interactive.
JavaScript helps create effects like animations and interactive elements. It uses features in web browsers to change how web pages look and work.
With JavaScript, you can use tools from companies like Disqus or Facebook on your website.
Many frameworks and libraries for JavaScript can speed up building websites and apps.
Start with a basic “Hello, World!” example. Add a “ tag to your HTML. You’ll learn to change text on your webpage.
JavaScript lets you declare variables with `let`, `const`, or `var`. They work in different ways. `let` and `const` are newer and better.
JavaScript has many operators for math, comparisons, and logic. They help with different tasks in coding.
Conditional statements let you make decisions in your code. They include the `if`, `else`, and `switch` statements for different cases.
Functions group code for specific tasks. They are a key part of breaking down a program into manageable parts.
JavaScript brings websites to life, adding interactivity. Knowing JavaScript well helps developers build amazing websites.
More:
- Master CSS Language: Tips for Stylish Web Design
- Master HTML Language – Create Stunning Web Pages
- Learn ASP Language: Key Benefits & Features
- Unlock the Power of PHP Language for Web Development
- Unlocking Potential with Python Language Skills
Leave a Reply